区块链的世界里,以太坊可是个明星级别的存在!而在这个明星平台上,智能合约更是闪耀的明珠。今天,就让我带你一起走进Go语言与以太坊智能合约的奇妙世界,一起探索这个充满无限可能的编程领域吧!
一、Go语言:简洁高效的编程利器
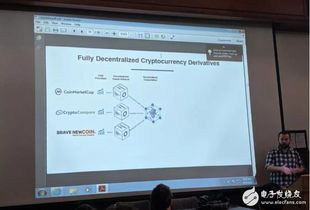
说到Go语言,你可能会想到它的简洁、高效和并发处理能力。没错,Go语言正是以太坊智能合约开发中的得力助手。它拥有丰富的库和工具,可以帮助开发者轻松地与以太坊区块链交互。
Go语言的特点如下:
1. 简洁明了:Go语言的语法简洁,易于阅读和理解,让开发者可以快速上手。
2. 并发处理:Go语言内置了并发处理机制,可以轻松实现高并发应用。
3. 跨平台:Go语言支持跨平台编译,可以在多种操作系统上运行。
二、以太坊智能合约:自动执行的合约
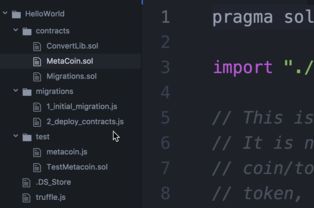
以太坊智能合约是一种自动执行的合约,它将合同条款直接写入代码中。当预设条件满足时,合约会自动执行相应的操作。这种去中心化的、自动化的特性,使得智能合约在金融、供应链、版权等多个领域具有广泛的应用前景。
以太坊智能合约的特点如下:
1. 去中心化:智能合约在区块链上运行,没有中央机构控制,所有节点共同维护网络。
2. 安全性:利用密码学技术保证交易的安全性,防止欺诈和篡改。
3. 可编程性:支持高级编程语言Solidity,可以编写复杂的智能合约。
三、Go语言与以太坊智能合约的融合
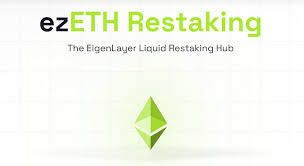
Go语言与以太坊智能合约的结合,为开发者带来了诸多便利。以下是一些使用Go语言开发以太坊智能合约的要点:
1. 安装Geth客户端:Geth是Go语言实现的以太坊客户端,可以用来连接以太坊网络、发送交易、部署智能合约等。
2. 使用Remix IDE:Remix是一个基于浏览器的智能合约开发环境,支持多种编程语言,包括Go语言。在Remix中,你可以编写、编译和部署Go语言编写的智能合约。
3. Solidity与Go语言的交互:Solidity是以太坊智能合约的主要编程语言,Go语言可以通过Web3.js库与Solidity合约进行交互。
四、Go语言开发以太坊智能合约的实践
以下是一个简单的Go语言智能合约示例,用于实现一个简单的代币发行系统:
```go
package main
import (
\t\fmt\
\t\math/big\
\t\github.com/ethereum/go-ethereum/common\
\t\github.com/ethereum/go-ethereum/contracts/abi/bind\
\t\github.com/ethereum/go-ethereum/core/types\
\t\github.com/ethereum/go-ethereum/ethclient\
// Token struct represents the Token smart contract
type Token struct {
\tAddress common.Address
\tbind.BoundContract
// NewToken creates a new instance of the Token struct
func NewToken(address common.Address, client bind.ContractBackend) (Token, error) {
\tcontract, err := bind.NewBoundContract(address, TokenABI, client)
\tif err != nil {
\t\treturn nil, err
\treturn &Token{address, contract}, nil
// TotalSupply returns the total supply of the token
func (t Token) TotalSupply() (big.Int, error) {
\treturn t.Call(nil, \totalSupply\)
// BalanceOf returns the balance of the specified address
func (t Token) BalanceOf(address common.Address) (big.Int, error) {
\treturn t.Call(nil, \balanceOf\, address)
// Transfer sends tokens from one address to another
func (t Token) Transfer(to common.Address, amount big.Int) (types.Transaction, error) {
\treturn t.Transact(bind.NewTransactOpts(common.Address{}, amount, nil), \transfer\, to, amount)
func main() {
\t// Connect to the Ethereum network
\tclient, err := ethclient.Dial(\https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID\)
\tif err != nil {
\t\tfmt.Println(\Failed to connect to Ethereum network:\, err)
\t\treturn
\t// Create a new instance of the Token struct
\ttoken, err := NewToken(common.HexToAddress(\0x...\), client)
\tif err != nil {
\t\tfmt.Println(\Failed to create Token instance:\, err)
\t\treturn
\t// Get the total supply of the token
\ttotalSupply, err := token.TotalSupply()
\tif err != nil {
\t\tfmt.Println(\Failed to get total supply:\, err)
\t\treturn
\tfmt.Printf(\Total supply: %s\
\, totalSupply.String())
\t// Get the balance of the specified address
\taddress := common.HexToAddress(\0x...\)
\tbalance, err := token.BalanceOf(address)
\tif err != nil {
\t\tfmt.Println(\Failed to get balance:\, err